Web APIs 认知
作用和分类
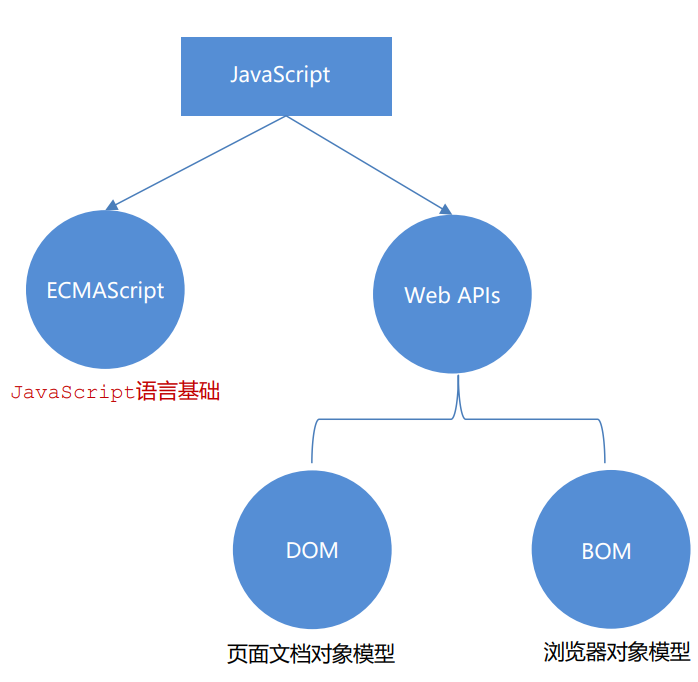
- 作用:就是使用JS去操作html和浏览器
- 分类:DOM(文档对象模型)、BOM(浏览器对象模型)
DOM
DOM(Document Object Model - 文档对象模型)是将整个 HTML 文档的每一个标签元素视为一个对象,这个对象下包含了许多的属性和方法,通过操作这些属性或者调用这些方法实现对 HTML 的动态更新,为实现网页特效以及用户交互提供技术支撑。
简单的说:DOM是浏览器提供的一套专门用来操作网页内容的功能
作用:开发网页内容特效和实现用户交互
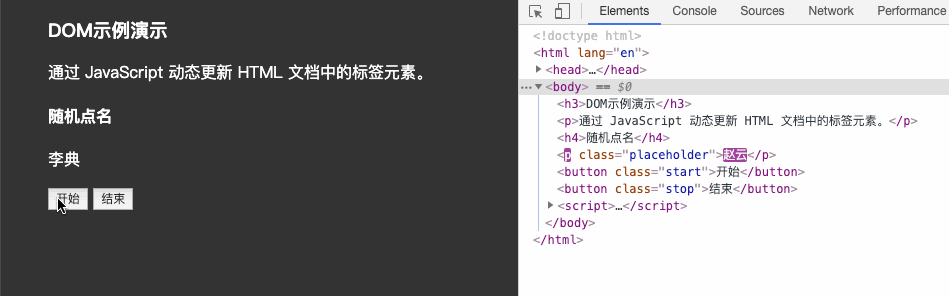
DOM树
- 将HTML文档以树状结构直观表现出来,称为文档树或DOM树
- 描述网页内容关系的名词
- 作用:直观地体现了标签与标签之间的关系
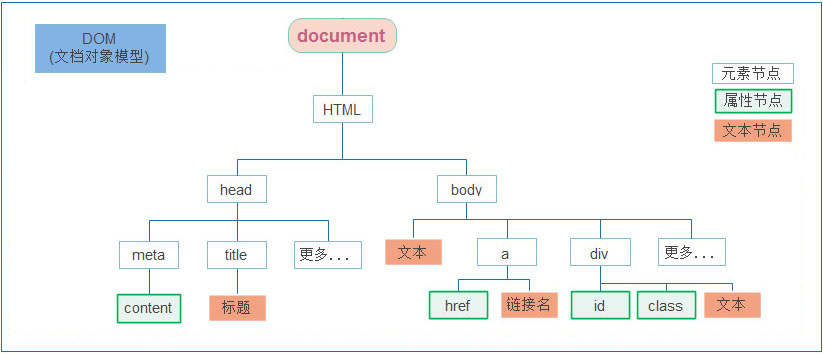
DOM对象
DOM对象:浏览器根据html标签生成的JS对象
- 所以的标签属性都可以在这个对象上找到
- 修改这个对象的属性会自动映射到标签上
document
document
是 DOM 里提供的一个对象,该对象包含了若干的属性和方法,document
是学习 DOM 的核心。
是用来访问和操作网页内容的
1 2 3 4 5 6 7 8 9 10 11
|
console.log(document.documentElement);
console.log(document.body);
document.write('Hello World!');
|
获取DOM元素
querySelector
满足条件(一个或多个CSS选择器)的第一个元素
querySelectorAll
满足条件(一个或多个CSS选择器)的元素集合,返回伪数组
- 伪数组:有长度和索引号的数组,但是没有
pop()
、push()
等方法
- 就算只有一个,也是返回一个伪数组
了解其他方式
getElementById
:根据id获取一个元素
getElementsByTagName
:根据标签获取一类元素
getElementByClassName
:根据类名获取元素
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>DOM - 查找节点</title> </head> <body> <h3>查找元素类型节点</h3> <p>从整个 DOM 树中查找 DOM 节点是学习 DOM 的第一个步骤。</p> <ul> <li>元素</li> <li>元素</li> <li>元素</li> <li>元素</li> </ul> <script> const p = document.querySelector('p') const lis = document.querySelectorAll('li') </script> </body> </html>
|
总结:
document.getElementById
专门获取元素类型节点,根据标签的 id
属性查找
- 任意 DOM 对象都包含
nodeType
属性,用来检检测节点类型
操作元素内容
通过修改 DOM 的文本内容,动态改变网页的内容。
innerText
将文本内容添加/更新到任意标签位置,文本中包含的标签不会被解析。
1 2 3 4 5 6
| <script> const intro = document.querySelector('.intro') intro.innerText = '嗨~ 我叫李雷!' </script>
|
innerHTML
将文本内容添加/更新到任意标签位置,文本中包含的标签会被解析。
1 2 3 4 5 6
| <script> const intro = document.querySelector('.intro') intro.innerHTML = '嗨~ 我叫韩梅梅!' intro.innerHTML = '<h4>嗨~ 我叫韩梅梅!</h4>' </script>
|
总结:如果文本内容中包含 html
标签时推荐使用 innerHTML
,否则建议使用 innerText
属性。
操作元素属性
操作元素常用属性
- 直接能过属性名修改,最简洁的语法
1 2 3 4 5 6 7 8
| <script> const pic = document.querySelector('.pic') pic.src = './images/lion.webp' pic.width = 400; pic.alt = '图片不见了...' </script>
|
操作元素样式属性
- 通过修改行内样式
style
属性,实现对样式的动态修改。
通过元素节点获得的 style
属性,本身的数据类型也是对象,如 box.style.color
、box.style.width
分别用来获取元素节点 CSS 样式的 color
和 width
的值。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>练习 - 修改样式</title> </head> <body> <div class="box">随便一些文本内容</div> <script> const box = document.querySelector('.intro') box.style.color = 'red' box.style.width = '300px' box.style.backgroundColor = 'pink' </script> </body> </html>
|
- 任何标签都有
style
属性,通过 style
属性可以动态更改网页标签的样式,如要遇到 css
属性中包含字符 -
时,使用驼峰命名法,要将 -
去掉并将其后面的字母改成大写,如 background-color
要写成 box.style.backgroundColor
- 操作类名(className) 操作CSS
如果修改的样式比较多,直接通过style属性修改比较繁琐,我们可以通过借助于css类名的形式。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>练习 - 修改样式</title> <style> .pink { background: pink; color: hotpink; } </style> </head> <body> <div class="box">随便一些文本内容</div> <script> const box = document.querySelector('.intro') box.className = 'pink' </script> </body> </html>
|
注意:
由于class是关键字, 所以使用className去代替
className是使用新值换旧值, 如果需要添加一个类,需要保留之前的类名
通过 classList 操作类控制CSS
为了解决className
容易覆盖以前的类名,我们可以通过classList
方式追加、删除和切换类名
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <style> div { width: 200px; height: 200px; background-color: pink; } .active { width: 300px; height: 300px; background-color: hotpink; margin-left: 100px; } </style> </head> <body> <div class="one"></div> <script> let box = document.querySelector('div') box.classList.add('active') box.classList.remove('one') box.classList.toggle('one') </script> </body> </html>
|
操作表单元素属性
表单很多情况,也需要修改属性,比如点击眼睛,可以看到密码,本质是把表单类型转换为文本框
正常的有属性有取值的跟其他的标签属性没有任何区别
获取:DOM对象.属性名
设置:DOM对象.属性名= 新值
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> <input type="text" value="请输入"> <button disabled>按钮</button> <input type="checkbox" name="" id="" class="agree"> <script> let input = document.querySelector('input') input.value = '小米手机' input.type = 'password' let btn = document.querySelector('button') btn.disabled = false let checkbox = document.querySelector('.agree') checkbox.checked = false </script> </body> </html>
|
自定义属性
标准属性: 标签天生自带的属性,比如class
、id
、title
等, 可以直接使用点语法操作,比如: disabled
、checked
、selected
自定义属性:
- 在html5中推出来了专门的data-自定义属性
- 在标签上一律以
data-
开头
- 在DOM对象上一律以
dataset
对象方式获取
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> <div data-id="1" data-name="自定义"> 自定义属性 </div> <script> let div = document.querySelector('div') console.log(div.dataset.id) console.log(div.dataset.name) </script> </body> </html>
|
定时器-间歇函数
定时器函数可以开启和关闭定时器
setInterval(函数, 间隔时间)
- 作用:每隔一段时间调用一次这个函数
- 间隔时间单位是毫秒ms
- 打开定时器
1 2 3 4 5 6 7 8
| <script> function repeat() { console.log('不知疲倦的执行下去....') } setInterval(repeat, 1000) </script>
|
- 关闭定时器
1 2 3 4 5 6
| let timer = setInterval(function() { console.log('执行下去!') }, 1000)
clearInterval(timer)
|